Comments in C are notes or explanations that people can read in the source code of a C program. A comment helps you read and understand the code better. Any compiler or interpreter will not run these lines.
It is recommended that we use notes to explain our code.
When and why should you use comments in C programming?
- If there are no comments about the features of the program, someone reading a long piece of code will get confused.
- You can add more information to code with comments in C. Comments make code easier to read.
- In C comments, you can explain a method so that the code is easier to understand.
- Comments in C can be used to stop certain parts of the code from running.
Types of comments in C
There are two kinds of comments in the C programming language:
- Single-line comment
- Multi-line comment
Single-line Comments in C
A comment in C is a single line that starts with //
. It begins and ends with the same line. As an example,
#include <stdio.h> int main(void) { // This is a single-line comment printf("Hello World!"); return 0; }
Output
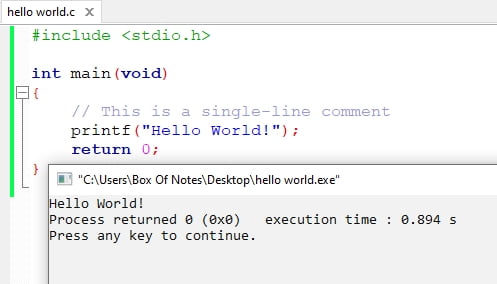
In the above example, // This is a single-line comment
is a single-line comment.
We can also use the single-line comment along with the code. For example,
printf("Hello World!"); // This is a single-line comment
Here, code before //
are executed and code after //
are ignored by the compiler.
Multi-line Comments in C
There is another type of comment in C code called a multi-line comment that lets us comment on more than one line at once.
The /*....*/
sign is used to write comments with multiple lines. As an example,
#include <stdio.h> int main(void) { /* The code below will print the words Hello World! to the screen, and it is amazing */ printf("Hello World!"); return 0; }
Output
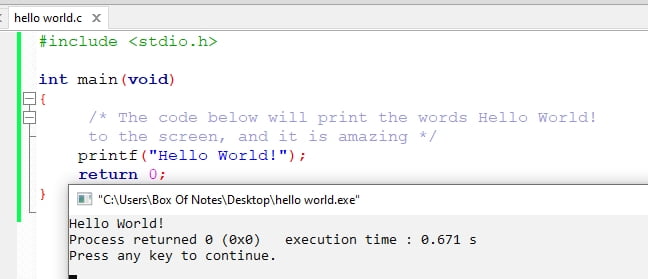
In this type of comment, the C compiler ignores everything from /*
to */
.
We have created a blog article on “Check if a given string is a comment or not with an Explanation“. You can check the article here.