In this tutorial, we will create a C Program to Check if a given string is a comment or not with explanation. Whether a given string or line is a comment or not we will check that with this program.
Types of comments in programs:
● Single Line Comment: Comments preceded by a Double Slash (‘//’)
● Multi-line Comment: Comments starting with (‘/*’) and ending with (‘*/’).
Code Explanation
The goal is to see if the given string is a comment. The procedure is as follows:
- Check if at the first Index(i.e. index 0) the value is ‘/’ then follow below steps else print “It is not a comment”.
- If line[0] == ‘/’:
- If line[1] == ‘/’, then print “It is a single line comment”.
- If line[1] == ‘*’, then traverse the string, and if any adjacent pair of ‘*’ & ‘/’ is found then print “It is a multi-line comment”.
- Otherwise, print “It is not a comment”.
Check if a Given String is a Comment or not with Explanation in C Program
The following is an example of how to put the aforesaid strategy into action:
#include<stdio.h> #include<string.h> int main() { char str[100]; int i=2,a=0; printf("Enter the String: \n"); scanf("%[^\n]s", &str); printf("\nOutput:\n"); if(str[0]=='/'){ if(str[1]=='/') printf("It is a Single Line comment\n"); else if(str[1]=='*'){ for(i=2;i<=100;i++){ if(str[i]=='*' && str[i+1]=='/'){ printf("It is a Multi-Line comment\n"); a=1; break; } else continue; } if(a==0){ printf("It is not a comment \n"); } } else printf("It is not a comment\n"); } else printf("It is not a comment\n"); }
Sample Input and Output
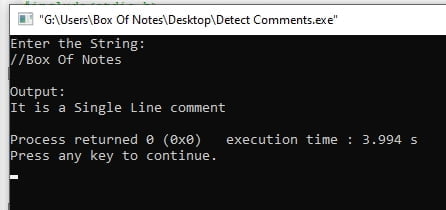
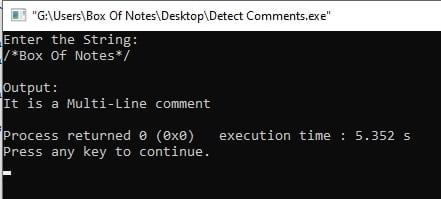