The escape sequence in C is the characters or the sequence of characters that can be used inside the string literal. The escape sequence is used to represent special characters that cannot be typed normally. Some characters in escape sequences are included in the ASCII character set, while others are not.
However, the result varies depending on the compiler you’re using, as different escape sequences represent different characters.
Escape Sequence List
The following table provides examples of frequent escape sequences in C language.
Escape Sequence | Name | Description |
---|---|---|
\a | Alarm or Beep | In C, it generates a bell sound. |
\b | Backspace | Moves the cursor one point backward. |
\n | New Line | Moves the cursor one point backwards. |
\r | Carriage Return | Cursor moves to line start. |
\t | Horizontal Tab | It adds whitespace left of the cursor and shifts it. |
\v | Vertical Tab | Inserts vertical space. |
\\ | Backlash | Inserts backslash. |
\’ | Single Quote | Displays a single quotation mark. |
\” | Double Quote | Displays double quote marks. |
\ooo | Octal Number | It represents octal numbers. |
\xhh | Hexadecimal Number | It represents hexadecimal numbers. |
\0 | NULL | It represents the NULL character. |
The most commonly used escape sequences are \n and \0. Programmers no longer employ escape sequences like \f and \a.
Escape Sequence in C Examples
These examples of escape sequences show you how to use different types of escape sequences in the C programming language.
Example to demonstrate how to use \a escape sequence in C
#include <stdio.h> int main() { printf("My mobile number " "is 0\a1\a2\a3\a4\a5\a6\a7\a8\a9\a"); return (0); }
Output
Example to demonstrate how to use \b escape sequence in C
#include <stdio.h> int main(void) { // \b - backspace character transfers // the cursor one character back with // or without deleting on different // compilers. printf("Hello \b\b\b\b\b\bHi World!"); return (0); }
Output
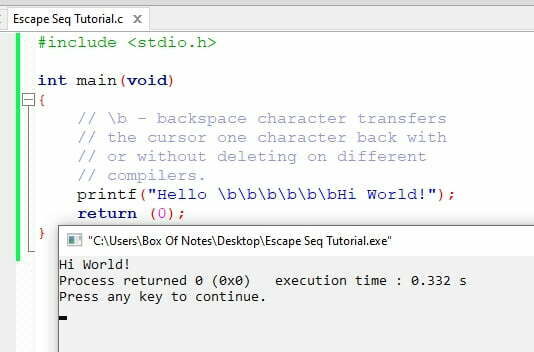
Example to demonstrate how to use \n escape sequence in C
#include <stdio.h> int main(void) { printf("Hello\n"); printf("World!"); return (0); }
Output
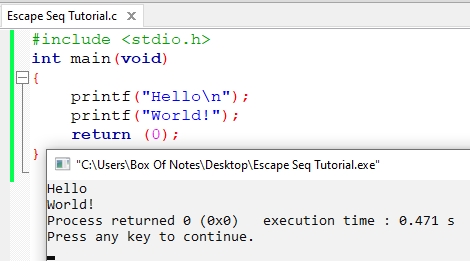
Example to demonstrate how to use \t escape sequence in C
#include <stdio.h> int main(void) { printf("Hello \t World!"); return (0); }
Output
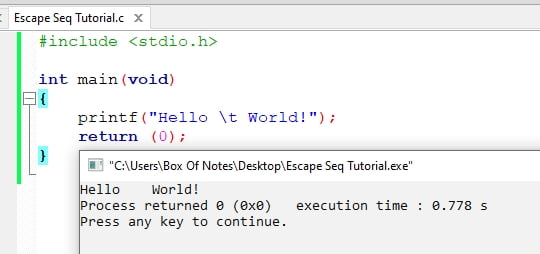
Example to demonstrate how to use \r escape sequence in C
#include <stdio.h> int main(void) { printf("Hello World \rBox Of Notes"); return (0); }
Output
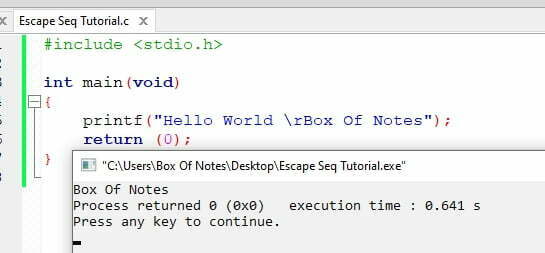
Example to demonstrate how to use \\ escape sequence in C
#include <stdio.h> int main(void) { printf("Hello\\World!"); return (0); }
Output
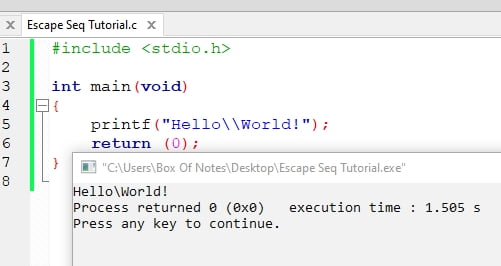
Example to demonstrate how to use \’ and \” escape sequence in C
#include <stdio.h> int main(void) { printf("\' Hello World \'\n"); printf("\" Hello World \""); return 0; }
Output
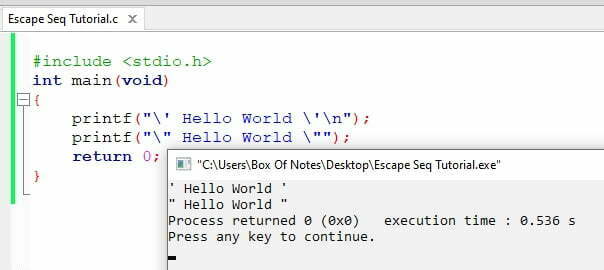
Example to demonstrate how to use \000 escape sequence in C
#include <stdio.h> int main(void) { // we are using \OOO escape sequence, here // each O in "OOO" is one to three octal // digits(0....7). char* s = "B\074\067"; printf("%s", s); return 0; }
Output
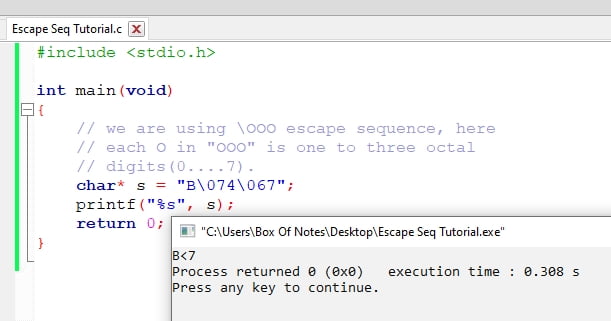
Explanation: 000 represents one to three octal digits (0-7) and requires at least one digit after \ and a maximum of three. First, convert 074 from octal to decimal, which is the ASCII value of char ‘<’. Instead of \072, the result is B<7.
Example to demonstrate how to use \xhh escape sequence in C
#include <stdio.h> int main(void) { // We are using \xhh escape sequence. // Here hh is one or more hexadecimal // digits(0....9, a...f, A...F). char* s = "B\x9f"; printf("%s", s); return 0; }
Output
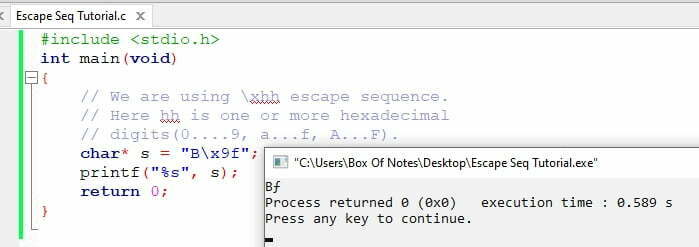
Explanation: Here, hh is a hexadecimal digit (0-9, a-f, A-F). More than one hexadecimal number can follow \x. The hexadecimal number ‘\x9f’ is used as a single character. Firstly it will get converted into decimal notation and it is the ASCII value of the char ‘ƒ’. Instead of \x9f, put ƒ. Hence, Bƒ output.