The popular “Hello World” program is where most people who want to learn a programming language begin. When run, this program displays “Hello World.” This easy example is meant to help you understand how C programs are put together and run
Program to Display “Hello World”
#include <stdio.h> int main() { printf("Hello World!"); return 0; }
Output
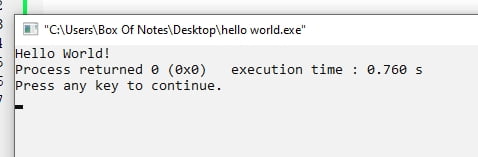
How Hello World program work?
#include
In the C program, lines that begin with “#” are called directives. The compiler calls the preprocessor program, which then handles these lines.
The #include
directive instructs the compiler to include a specific file, and the #include<stdio.h>
directive instructs the compiler to include the header file for the Standard Input Output file. This file contains declarations of all of the standard input/output library functions.
int main()
We use this line to define a function called “main” that gives back integer-type data. There is a bunch of statements called a function that work together to do a certain job. The main() method is where all C programs start running, no matter where it is in the program. That’s why every C program needs to have a main() function. This is where the program starts to run.
{ and }: The starting braces “{” start the main function, and the closing braces “}” end it. The body of the main function is made up of everything between these two points. These points are called blocks.
printf("Hello World");
The compiler is told to show the word “Hello World” on the screen by this line. In C, this line is known as a statement. Each statement is meant to do something. A semicolon ‘;’ is used to end a statement. As a statement comes to an end, the semicolon character is used to show that the statement is over.
You can print a string of characters to the stdout console with the printf() function. The output device shows everything inside ” “.
return 0;
This is also a statement. When you use this statement, a function will return a value and the function will be over. In functions, such a statement is mostly used to return the results of the work that the function did.