In this tutorial, we will learn to create a chatbot in python in two different approaches. One is a simple basic chatbot using Dictionary and the other is with using the ChatterBot module.
A chatbot is a conversational application that replaces or augments human support agents with artificial intelligence (AI) and other automation technologies that can converse with end-users through chat.
Chatbot in Python using Dictionary
Dictionary in Python is a collection of keys and values, used to store data values like a map, which, unlike other data types holds only a single value as an element.
Dictionary holds the key: value pair. Key-Value is provided in the dictionary to make it more optimized.
Program
print("*............................................*") qna = { "hi": "Hello", "how are you?": "I am fine, what about you?", "who are you?": "I am no one. But who are you again??", "exit": "exit" } res = list(qna.keys()) for qs in res: print(f"-> {qs}") print("*............................................*") print("Enter your Question from Above...\n\n") while True: try: qs=input().lower() if(qs=='exit'): break else: print('Bot: '+qna[qs]) except: print("Bot: I don't know the answer you are looking for, Sorry!!!")
Input & Output
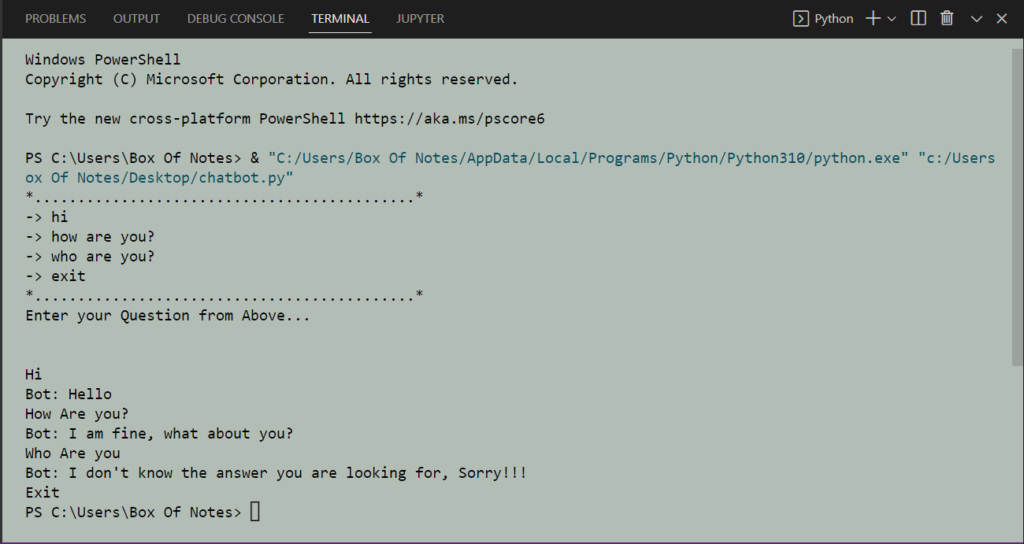
Explanation
At first we are creating a dictionary called qna
for storing keys and values.
Then get the dictionary keys in a list and then print them for users, to know which questions answer can the bot give.
Then creating a while loop. And generating user input into lower characters to match the dictionary keys.
We are using Try and Except because, if the user input is not in the dictionary then it will show a message which will be set by the programmer.
If we don’t use Try and Except, when the user types something which is not in the dictionary then it will show an error.
Chatbot in Python Using ChatterBot
Understanding the ChatterBot Library
ChatterBot is a Python library designed to respond to user inputs automatically. It employs a combination of Machine Learning algorithms to generate various types of responses. This feature allows developers to create Python chatbots that can converse with humans and provide relevant and appropriate responses. Furthermore, the ML algorithms assist the bot in improving its performance over time.
Language independence is another amazing feature of the ChatterBot library. The library is designed in such a way that the bot can be trained in more than one programming language.
Understanding the working of the ChatterBot library
When a user enters a specific input into the chatbot (created with ChatterBot), the bot saves both the input and the response for future use. This data (accumulated experiences) enables the chatbot to generate automated responses whenever a new input is fed into it.
The program selects the most relevant response from the nearest statement that matches the input and then delivers a response from the previously known set of statements and responses. As the chatbot engages in more communications, the precision of its responses improves.
Installation
Install chatterbot using Python Package Index(PyPi) with this command
pip install chatterbot
Some may get ModuleNotFound Error: No module named ‘pytz’ error. If you face the same issue then install the pytz like down below.
pip install pytz
Below is the implementation.
Program
from chatterbot import ChatBot from chatterbot.conversation import Statement from chatterbot.trainers import ChatterBotCorpusTrainer bot = ChatBot('ChatBot') trainer = ChatterBotCorpusTrainer(bot) trainer.train("chatterbot.corpus.english") print("Hello, I am your persoal ChatBot!!!") while True: qs = input(">>>") print(bot.get_response(Statement(text=qs, search_text=qs)))
Input & Output
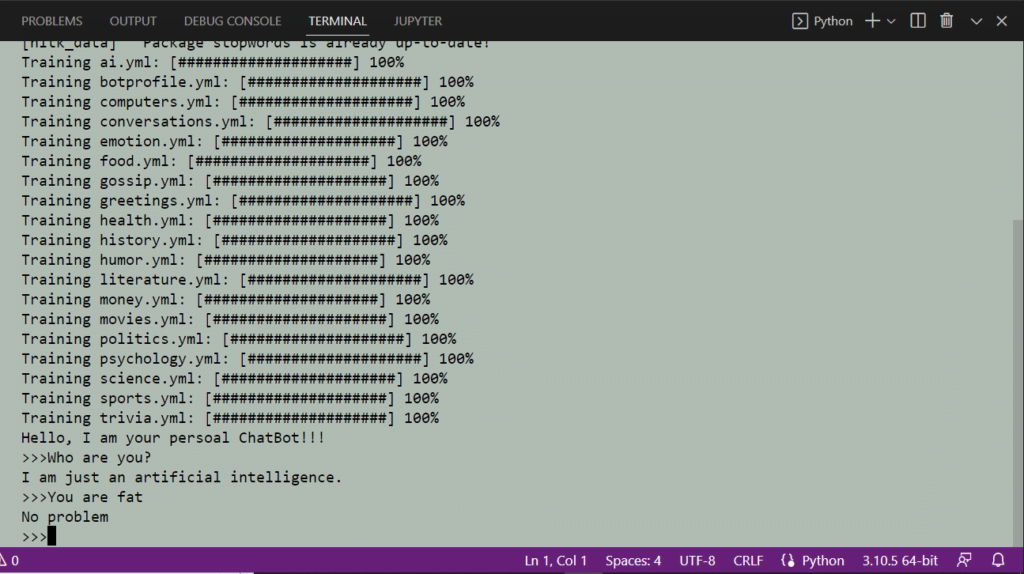
Explanation
First, we are importing chatbot from chatterbot.
Then we are importing statements from chatterbot conversion to get rid of the unnecessary warning messages.
Then we are importing a trainer from chatterbot based on the corpus.
A corpus is a collection of authentic text or audio organized into datasets.
We are giving our chatbot a name and storing it in a variable called bot.
Now we have to train the chatbot with the corpus trainer. So, we are creating a trainer for our chatbot, and whatever object we’re getting storing in one variable called the trainer.
We are using the training method to train the bot in the English Language from the corpus.
Then we are creating an infinite while loop to use the bot, we are taking input from the user as a statement to not generate an unwanted warning message and the bot generated its answer.
Advanced Method
There are so many languages you can train your bot with. If you want to train your bot in another language then train the bot in that language. Here is the list of language Chatterbot now have:
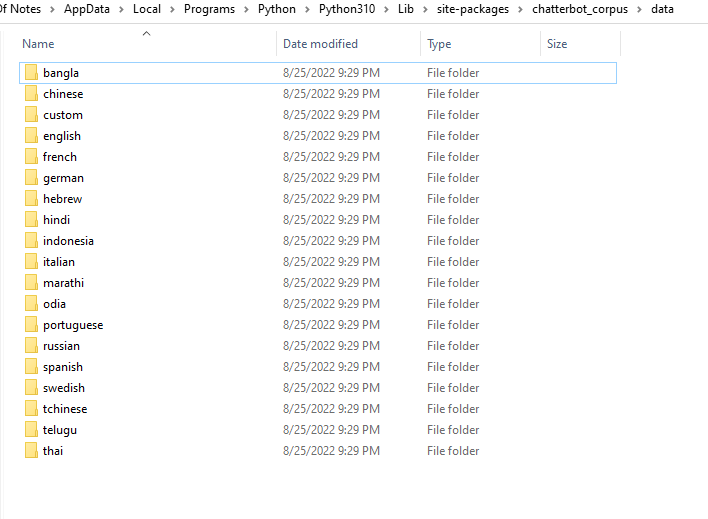
If you want to train your bot specifically in one topic, then add the filename at the last like down below:
trainer.train("chatterbot.corpus.english.greetings")
Here are the topics list chatterbot has for the English Language.
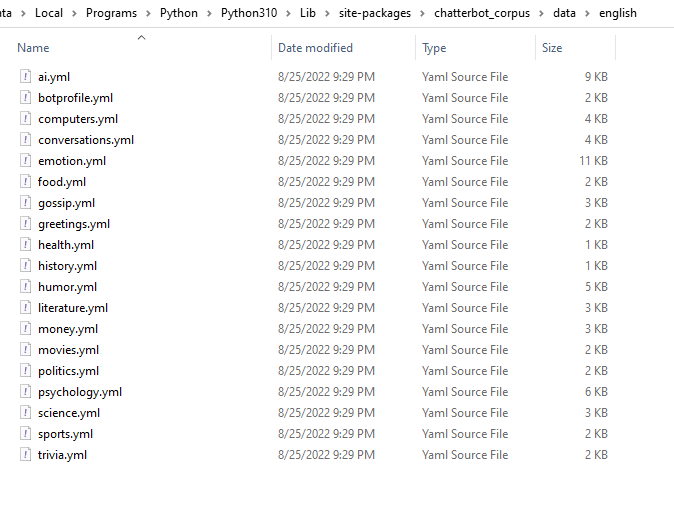
If you want to create your own yml file and then want to train your bot with that yml file, then do the following
First get the path or file location of the yml file. For example, we are storing psychology.yml in a desktop and getting the file location like these.
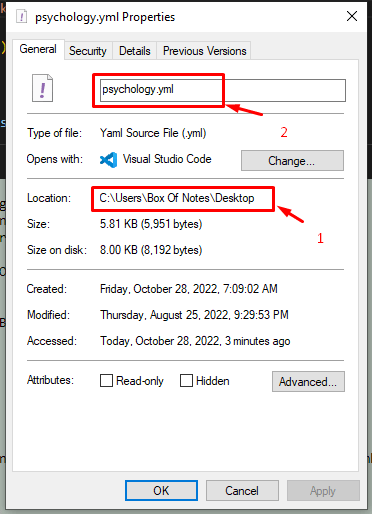
Copy these two and add them to the training method like down below. Remember, we have to add an extra backslash.
trainer.train("C:\\Users\\Box Of Notes\\Desktop\\psychology.yml")